Displaying Time and Date through JavaScript
I'm gonna start off by guessing you all know how to start off JavaScript - with <script language="JavaScript"></script> for all you people who don't know.
Date
Well inside the <script> tags for the basic date in an alert put this:
That will give you:
Now of course not everyone wants that do they? So that's when it get's an awful lot more complicated.
And that will give you this:
Time
If you want a basic (I say basic but it's rather hard!) time information alert you'll want this:
That will give you this:
JavaScript Clock
The script below displays the time according to your computer in an HTML form text input.
That code will give you this (with the current time):
I Hope This is of some use to you. Remember! JavaScript is case sensitive so be very careful. Also try not to delete any of the brackets or semi-colons or the scripts won't work.
I'm gonna start off by guessing you all know how to start off JavaScript - with <script language="JavaScript"></script> for all you people who don't know.
Date
Well inside the <script> tags for the basic date in an alert put this:
Code:
<script language="JavaScript">
var now = new Date();
alert( now );
</script>
That will give you:
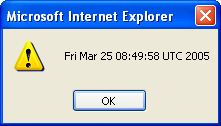
Now of course not everyone wants that do they? So that's when it get's an awful lot more complicated.
Code:
<script language="JavaScript">
var days = new Array("Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday");
var mons = new Array("January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December");
var now=new Date();
var yy = now.getYear();
varmm = now.getMonth(); mm=mons[mm];
var dd = now.getDate();
var dy = now.getDay(); day=days[dy];
alert(dy+" "+dd+" "+mm+" "+yy);
</script>
And that will give you this:
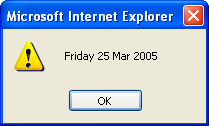
Time
If you want a basic (I say basic but it's rather hard!) time information alert you'll want this:
Code:
<script language="JavaScript">
var now = new Date();
var hh = now.getHours();
var mn = now.getMinutes();
var ss = now.getSeconds();
var ms = now.getMilliseconds();
var hi = "Good Morning";
if( hh > 11 ) hi= "Good Afternoon";
if( hh > 17 ) hi= "Good Evening";
var tim = hi + "\n";
tim += "Hours: " +hh+ "\n";
tim += "Minutes: " +mn+ "\n";
tim += "Seconds: " +ss+ "." +ms;
alert(tim);
</script>
That will give you this:
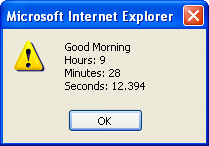
JavaScript Clock
The script below displays the time according to your computer in an HTML form text input.
Code:
<body onLoad="tick()">
<script language="JavaScript">
<!--
function tick(){
var now = new Date();
var hh = now.getHours(); if( hh <= 9 ) hh = "0" + hh;
var mn = now.getMinutes(); if( mn<= 9 ) mn = "0" + mn;
var ss = now.getSeconds(); if( ss <= 9 ) ss = "0" + mn;
var tt = hh + ": " +mn+ ": " + ss;
document.f.clock.value = tt;
window.setTimeout( "tick()", 1000 );
}
// -->
</script>
<form name="f">
<input name="clock" type="text" size="10">
</form>
</body>
That code will give you this (with the current time):

I Hope This is of some use to you. Remember! JavaScript is case sensitive so be very careful. Also try not to delete any of the brackets or semi-colons or the scripts won't work.
Last edited: